How to Create a Chat App in Android: A Comprehensive Guide
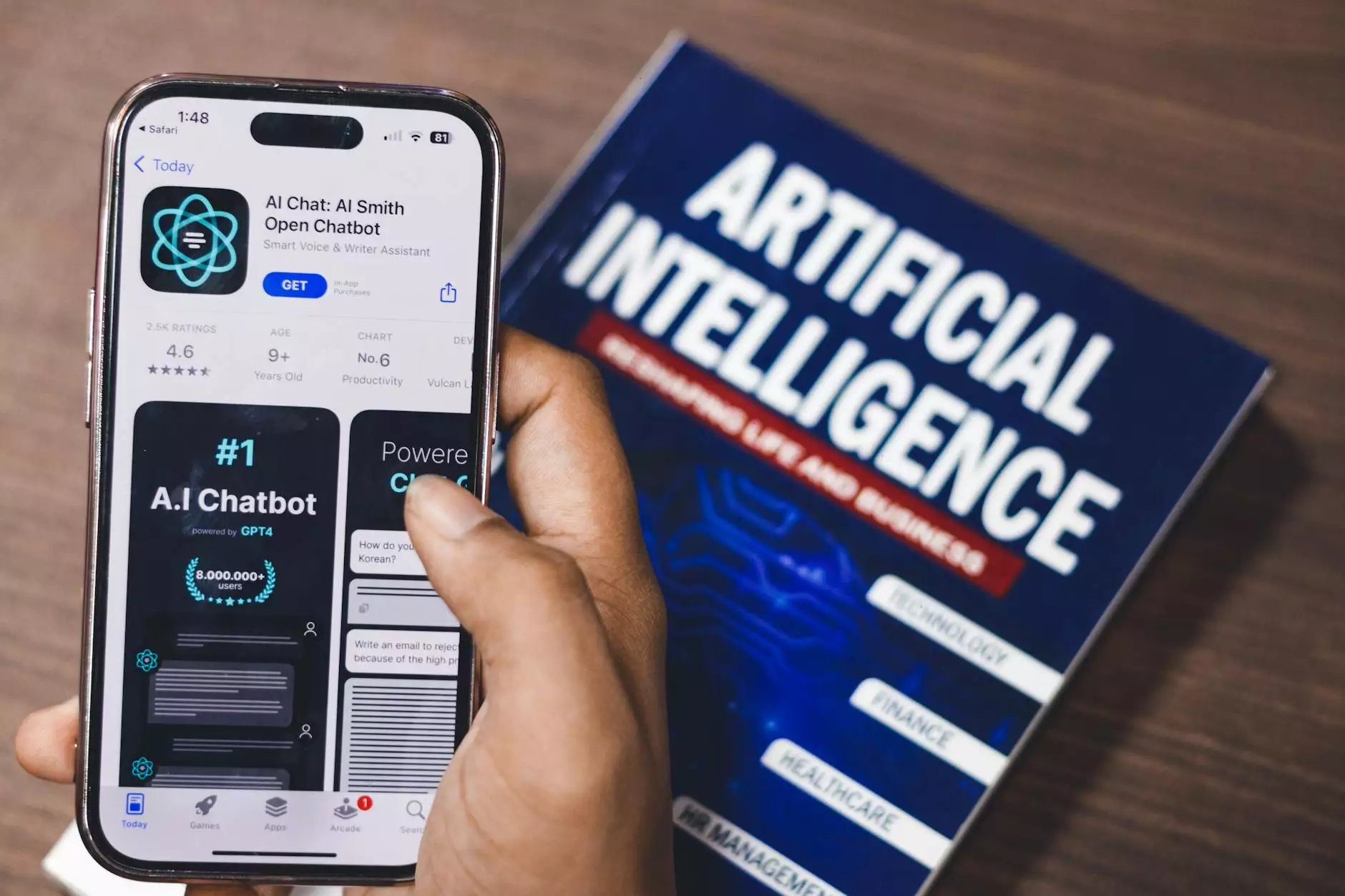
In today's fast-paced digital world, instant messaging is an integral part of our day-to-day communication. With millions of users relying on chat applications, building your own chat app in Android can be a rewarding endeavor. In this article, we will walk you through the essential steps to create a fully functional chat app for Android devices.
Understanding the Basics of a Chat Application
Before diving into the technical aspects, it's crucial to understand the fundamental components of a chat application. A typical chat app consists of the following features:
- User Authentication: Allows users to sign up and log in securely.
- Real-time Messaging: Enables instant message delivery between users.
- Media Sharing: Facilitates sending and receiving images, videos, and other files.
- Push Notifications: Notifies users of new messages even when the app is closed.
- User Profiles: Allows users to manage their personal information and settings.
Step 1: Setting Up Your Development Environment
To begin your journey in creating a chat app in Android, you must set up the necessary tools and frameworks. Here’s what you need:
- Android Studio: The official Integrated Development Environment (IDE) for Android development. Download it from the official website.
- Java or Kotlin: Choose between these two programming languages to build your chat application. Kotlin is the preferred choice for modern Android development.
- Firebase: Google’s mobile and web application development platform provides several tools, including authentication, real-time database, and cloud storage.
Step 2: Creating a New Android Project
Once your development environment is ready, the next step is to create a new Android project:
- Open Android Studio and click on Start a new Android Studio project.
- Select Empty Activity and click Next.
- Name your project and choose the language (Java or Kotlin).
- Set the Minimum API level, then click Finish.
Step 3: Implementing User Authentication
For any chat application, user authentication is crucial. Firebase Authentication provides an easy way to implement sign-up and login features:
- Add the Firebase SDK to your project by including it in your project-level build.gradle file.
- In your app-level build.gradle file, add the required dependencies for Firebase Authentication: implementation 'com.google.firebase:firebase-auth:latest_version'
- Initialize Firebase in your application: FirebaseApp.initializeApp(this);
- Use Email/Password authentication or Phone Number authentication as per your requirement.
Step 4: Building the User Interface
The user interface (UI) of your chat app is incredibly important for the user experience. Create a simple yet functional layout:
- Login and Registration Screens: Design user-friendly forms for authentication.
- Main Chat Screen: Use a RecyclerView to display chat messages.
- Message Input Box: Allow users to type and send messages directly from the main chat screen.
Example XML Layout for Main Activity
Step 5: Implementing Real-Time Messaging
Real-time messaging is the heart of any chat application. Firebase Realtime Database is an excellent choice for this function:
- Add the Firebase Realtime Database dependency in your app-level build.gradle file: implementation 'com.google.firebase:firebase-database:latest_version'
- Create a message data model: public class Message { private String userId; private String messageText; private String timeStamp; // Getters and Setters }
- Send messages to the Firebase database: DatabaseReference databaseReference = FirebaseDatabase.getInstance().getReference("messages"); Message message = new Message(userId, messageText, timeStamp); databaseReference.push().setValue(message);
- Retrieve messages in real-time using a listener: databaseReference.addValueEventListener(new ValueEventListener() { @Override public void onDataChange(DataSnapshot dataSnapshot) { // Handle incoming messages } @Override public void onCancelled(DatabaseError databaseError) { // Handle error } });
Step 6: Media Sharing Functionality
Allowing users to share images and media files enhances the chat experience. You can implement this using the following steps:
- Implement the image picker library to choose images: implementation 'com.github.yalantis:ucrop:latest_version'
- Use Intent to start an image selection activity: Intent intent = new Intent(Intent.ACTION_PICK, MediaStore.Images.Media.EXTERNAL_CONTENT_URI); startActivityForResult(intent, REQUEST_IMAGE_PICK);
- Upload the selected image to Firebase Storage and retrieve the download URL: StorageReference storageReference = FirebaseStorage.getInstance().getReference("images"); storageReference.child(imageName).putFile(imageUri).addOnSuccessListener(taskSnapshot -> { // Get download URL and send it as a message });
Step 7: Enabling Push Notifications
Staying connected with users is vital in a chat application. Implementing push notifications ensures that users are notified of new messages:
- Add Firebase Cloud Messaging to your project: implementation 'com.google.firebase:firebase-messaging:latest_version'
- Set up Firebase Messaging in your application: public class MyFirebaseMessagingService extends FirebaseMessagingService { @Override public void onMessageReceived(RemoteMessage remoteMessage) { // Handle incoming messages } }
- Send notifications from your server or Firebase console when a new message is received.
Step 8: Testing and Debugging
Thorough testing is vital to ensure that your chat app is functional and user-friendly. Utilize the following techniques:
- Unit Testing: Write unit tests for individual components.
- User Acceptance Testing: Get feedback from potential users and iterate based on their input.
- Debugging Tools: Use Android Studio's Logcat and debugging features to identify and fix issues.
Conclusion
Creating a chat app in Android can be an enjoyable and educational experience. By following the steps outlined in this guide, you can build a robust, real-time messaging application that meets modern user expectations. Remember to keep your code clean, implement user feedback, and continue enhancing your app with new features. Happy coding!
Get Started Today
If you’re inspired to take the plunge into Android development, visit nandbox.com for more resources and tools that can help you innovate in the mobile world. Whether it’s through no-code solutions or tailored software development services, the possibilities are limitless!
how to create a chat app in android